There are several images on a web page. You need to:
- get the number of all images on the page
- process each image (for example, get its location)
Also, I'm going to explain QTP Descriptive Programming concepts.
Let's start with Google Book Search page:
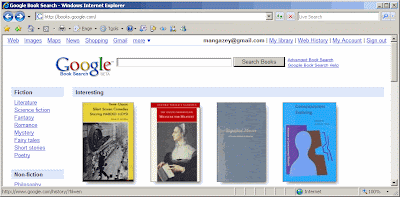
I have recorded simple QTP script which:
- uses http://books.google.com as initial URL
- clicks 'Google Book Search' image:
Browser("Google Book Search").Page("Google Book Search").Image("Google Book Search").Click
Why have I recorded this script?
Because it will help us to investigate QTP Object Repository (OR) and properties of image.
Let's open Object Repository:
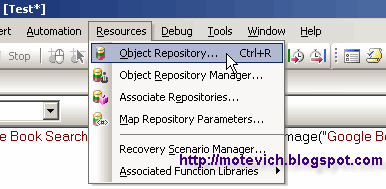
Using "html tag" property and its value "IMG", we can create general description for all images on the page. For that I use QTP Description object.
Description object is used to create a 'Properties' collection object. Each 'Property' object contains a property name and value pair.
Set descImage = Description.Create
descImage("html tag").value = "IMG"
The above code:descImage("html tag").value = "IMG"
- creates Description object
- creates "html tag" property and sets its value to "IMG"
You can use the following code to get number of all images on a Web page:
Dim descImage, listImages
' Create description for all images on a Web page.
' For that we use "html tag" property and its value "IMG"
Set descImage = Description.Create
descImage("html tag").value = "IMG"
' Get all images which match the above description
Set listImages = Browser("Google Book Search").Page("Google Book Search").ChildObjects(descImage)
' Show the number of found images
MsgBox "Found images: " & listImages.Count
' Create description for all images on a Web page.
' For that we use "html tag" property and its value "IMG"
Set descImage = Description.Create
descImage("html tag").value = "IMG"
' Get all images which match the above description
Set listImages = Browser("Google Book Search").Page("Google Book Search").ChildObjects(descImage)
' Show the number of found images
MsgBox "Found images: " & listImages.Count
Execute the above code and you will get a result like:
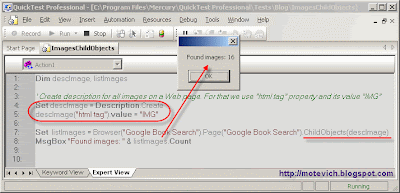
How does Description object work?
Actually, QTP Description object is a part of QTP Descriptive Programming.QTP Descriptive Programming (DP) is a way of working with objects without Object Repository (OR).
In our example, we couldn't know all images on a Web page in advance. So, we couldn't add them into QTP Object Repository. Instead of that, we created Description object with required property and its value.
Tips: We can use several properties assigned to Description object. For example:
Set descImage = Description.Create
descImage("html tag").value = "IMG"
descImage("image type").value = "Image Link"
descImage("html tag").value = "IMG"
descImage("image type").value = "Image Link"
When we pass Description object to ChildObjects QTP function, it returns the collection of child objects contained within the object (Page("Google Book Search")) and matched to Description object.
That's why we've got the list of all images on a page.
How to get additional properties of found images?
As you remember, we planned to process each image (get its location).
Let's open OR and check the additional recorded properties:
There are "src" property, which contains the URL address of the image file.
To get this property, we can use GetROProperty QTP function.
The final code is:
Dim descImage, listImages
' Create description for all images on a Web page.
' For that we use "html tag" property and its value "IMG"
Set descImage = Description.Create
descImage("html tag").value = "IMG"
' Get all images which match the above description
Set listImages = Browser("Google Book Search").Page("Google Book Search").ChildObjects(descImage)
' Show the number of found images
MsgBox "Found images: " & listImages.Count
' Show location ("src" property) of each image
For i = 0 To listImages.Count - 1
MsgBox "Image #:" & (i+1) & ": " & listImages(i).GetROProperty("src")
Next
' Create description for all images on a Web page.
' For that we use "html tag" property and its value "IMG"
Set descImage = Description.Create
descImage("html tag").value = "IMG"
' Get all images which match the above description
Set listImages = Browser("Google Book Search").Page("Google Book Search").ChildObjects(descImage)
' Show the number of found images
MsgBox "Found images: " & listImages.Count
' Show location ("src" property) of each image
For i = 0 To listImages.Count - 1
MsgBox "Image #:" & (i+1) & ": " & listImages(i).GetROProperty("src")
Next
This code shows a location of each image from a Web page.
There is a message box with a location of first image:
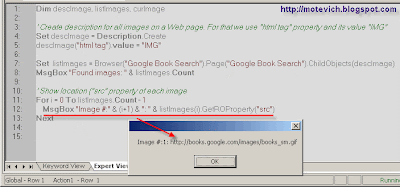
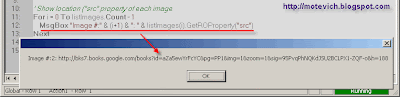
Tips: Use the table of the most popular properties of Image QTP control:
Tips: You can use the same approach to work with others UI controls - Links, WebEdit, WebList, etc.
Related articles:
- QTP Descriptive Programming - How to get number of objects
- QTP Descriptive Programming - How to perform operations on objects
- QTP Descriptive Programming - How to close browsers
- QTP - How to capture tool tip?
- QTP VIDEO - How to run QTP test from command line
- How to minimize/maximize QTP window before the QTP script execution
- All QTP visual tutorials
Dear readers,
Do you have interesting thoughts/articles/notes on automated testing?
Please, feel free to send them. Join our team!
--
Dmitry Motevich
14 comments:
The content presented for descriptive programming is good. in Future can we expect batch testing and driver scripts and recovery scenarios.
Regards,
Vinesh
2Satyam:
What do you mean with "batch testing"?
hi dmitry,
I worked your image exercise its really good. But i have one problem while i try to record the flash object.In OR
description value
windowid 105139264
regexpwndclass MacromediaFlashPlayerActiveX
like that created
During running:
It shows general run error.
my question is how to identify all flash objects and its "src"?
please mail me:johnsonraja@gmail.com
@johnson,
I'm afraid I cannot help you.
I've never worked with Flash from QTP.
Hi Dmitry,
I am trying to click on one image link in my web application.But all the time QTP can not identify that object. I have added image type, alt, html tag properties to image.After descriptive programming also its not clicking on that image.
Plz let me know if u have any idea.
Thanks!
Anon
how to handle image link in descriptive programing
@revaraghavan,
Explained in others articles.
Search this blog.
Hi
It was very useful for my practice.
Can you solve one more problem for me.
In a webpage, there is a webtable which has 15 identical images. I have used Description.create method and used src property as per your example.
Then i used a for loop for clicking that image and coming back again to that page. After navigating back to the page QTP is not identifying the image and throws run error.
QTP is executing for the first iteration in the for loop and it fails for the second iteration.
If the second iteration value is given then the for loop executes correctly and again fails for the 3rd iteration.
The Content given in ur blog is really very nice.... it really helped me a lot
But i didnt found anything related to checking of recently added (runtime) data
for eg:-
I have to add admin users and after adding it is displayed on same page ..i need to add the admin and check if added admin user is displayed correclty or not.
could u please help me in creating script for checking above
@sangeeta268 (January 5, 2009),
You can use Output value. It's explained here:
QTP VIDEO - How to capture dynamic text?
hi the code which is given by you is working fine at my end but i want to print name of all books and nuber of books according to name of book,so how can i do this can u please guide me?
Dmitry - This is good information, thanks for posting it.
I did get your example to run but I'm confused about one point. The script seems to work only when the Google Book Search image is in the repository. If I remove this from the repository, I get an error on the line:
Set listImages = Browser("Google Book Search").Page("Google Book Search").ChildObjects(descImage)
Perhaps I am not quite clear on where the OR leaves off and descriptive programming takes over. Or perhaps I'm doing something wrong. If you can clarify for me it would be greatly appreciated.
Dmitry - This is good information, thanks for posting it.
I did get your example to run but I'm confused about one point. The script seems to work only when the Google Book Search image is in the repository. If I remove this from the repository, I get an error on the line:
Set listImages = Browser("Google Book Search").Page("Google Book Search").ChildObjects(descImage)
Perhaps I am not quite clear on where the OR leaves off and descriptive programming takes over. Or perhaps I'm doing something wrong. If you can clarify for me it would be greatly appreciated.
how to automate scroll bars and check the word wrap functioality
Post a Comment